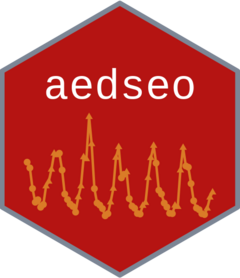
Compute seasonal onset and burden levels from seasonal time series observations.
Source:R/combined_seasonal_output.R
combined_seasonal_output.Rd
This function performs automated and early detection of seasonal epidemic onsets and estimates the burden levels from time series dataset stratified by season. The seasonal onset estimates growth rates for consecutive time intervals and calculates the sum of cases. The burden levels use the previous seasons to estimate the levels of the current season.
Arguments
- tsd
An object containing time series data with 'time' and 'observation.'
- disease_threshold
An integer specifying the threshold for considering a disease outbreak. For seasonal onset it defines the per time-step disease threshold that has to be surpassed to possibly trigger a seasonal onset alarm. If the total number of cases in a window of size k exceeds
disease_threshold * k
, a seasonal onset alarm can be triggered. For burden levels it defines the per time-step disease threshold that has to be surpassed for the observation to be included in the level calculations.- family
A character string specifying the family for modeling seasonal onset.
- family_quant
A character string specifying the family for modeling burden levels.
- season_start, season_end
Integers giving the start and end weeks of the seasons to stratify the observations by.
- only_current_season
Should the output only include results for the current season?
- ...
Arguments passed to
seasonal_burden_levels()
,fit_percentiles()
andseasonal_onset()
functions.
Value
An object containing two lists: onset_output and burden_output:
onset_output:
A seasonal_onset
object containing:
'reference_time': The time point for which the growth rate is estimated.
'observation': The observation in the reference time point.
'season': The stratification of observables in corresponding seasons.
'growth_rate': The estimated growth rate.
'lower_growth_rate': The lower bound of the growth rate's confidence interval.
'upper_growth_rate': The upper bound of the growth rate's confidence interval.
'growth_warning': Logical. Is the growth rate significantly higher than zero?
'sum_of_cases': The sum of cases within the time window.
'sum_of_cases_warning': Logical. Does the Sum of Cases exceed the disease threshold?
'seasonal_onset_alarm': Logical. Is there a seasonal onset alarm?
'skipped_window': Logical. Was the window skipped due to missing?
'converged': Logical. Was the IWLS judged to have converged? - 'seasonal_onset': Logical. The first detected seasonal onset in the season?
burden_output:
A list containing:
'season': The season that burden levels are calculated for.
'high_conf_level': (only for intensity_level method) The conf_level chosen for the high level.
'conf_levels': (only for peak_level method) The conf_levels chosen to fit the 'low', 'medium', 'high' levels.
'values': A named vector with values for 'very low', 'low', 'medium', 'high' levels.
'par': The fit parameters for the chosen family.
par_1:
For 'weibull': Shape parameter.
For 'lnorm': Mean of the log-transformed observations.
For 'exp': Rate parameter.
'par_2':
For 'weibull': Scale parameter.
For 'lnorm': Standard deviation of the log-transformed observations.
For 'exp': Not applicable (set to NA).
'obj_value': The value of the objective function - (negative log-likelihood), which represent the minimized objective function value from the optimisation. Smaller value equals better optimisation.
'converged': Logical. TRUE if the optimisation converged.
'family': The distribution family used for the optimization.
'weibull': Uses the Weibull distribution for fitting.
'lnorm': Uses the Log-normal distribution for fitting.
'exp': Uses the Exponential distribution for fitting.
'disease_threshold': The input disease threshold, which is also the very low level.
Examples
# Generate random flu season
generate_flu_season <- function(start = 1, end = 1000) {
random_increasing_obs <- round(sort(runif(24, min = start, max = end)))
random_decreasing_obs <- round(rev(random_increasing_obs))
# Generate peak numbers
add_to_max <- c(50, 100, 200, 100)
peak <- add_to_max + max(random_increasing_obs)
# Combine into a single observations sequence
observations <- c(random_increasing_obs, peak, random_decreasing_obs)
return(observations)
}
season_1 <- generate_flu_season()
season_2 <- generate_flu_season()
start_date <- as.Date("2022-05-29")
end_date <- as.Date("2024-05-20")
weekly_dates <- seq.Date(from = start_date,
to = end_date,
by = "week")
tsd_data <- tsd(
observation = c(season_1, season_2),
time = as.Date(weekly_dates),
time_interval = "week"
)
#> Warning: `tsd()` was deprecated in aedseo 0.1.2.
#> ℹ Please use `to_time_series()` instead.
# Run the main function
combined_data <- combined_seasonal_output(tsd_data)
# Print seasonal onset results
print(combined_data$onset_output)
#> # A tibble: 52 × 13
#> reference_time observation season growth_rate lower_growth_rate
#> <date> <dbl> <chr> <dbl> <dbl>
#> 1 2023-05-28 6 2023/2024 -0.427 -0.501
#> 2 2023-06-04 81 2023/2024 -0.156 -0.232
#> 3 2023-06-11 121 2023/2024 0.0834 0.0120
#> 4 2023-06-18 145 2023/2024 0.287 0.217
#> 5 2023-06-25 157 2023/2024 0.381 0.314
#> 6 2023-07-02 199 2023/2024 0.197 0.143
#> 7 2023-07-09 237 2023/2024 0.169 0.121
#> 8 2023-07-16 385 2023/2024 0.256 0.213
#> 9 2023-07-23 392 2023/2024 0.246 0.207
#> 10 2023-07-30 430 2023/2024 0.191 0.156
#> # ℹ 42 more rows
#> # ℹ 8 more variables: upper_growth_rate <dbl>, growth_warning <lgl>,
#> # sum_of_cases <dbl>, sum_of_cases_warning <lgl>, seasonal_onset_alarm <lgl>,
#> # skipped_window <lgl>, converged <lgl>, seasonal_onset <lgl>
# Print burden level results
print(combined_data$burden_output)
#> $season
#> [1] "2023/2024"
#>
#> $values
#> very low low medium high
#> 20.00000 77.41445 299.64984 1159.86133
#>
#> $optim
#> $optim$par
#> [1] 6.94863952 0.06530442
#>
#> $optim$obj_value
#> [1] 33.83299
#>
#> $optim$converged
#> [1] TRUE
#>
#> $optim$high_conf_level
#> [1] 0.95
#>
#> $optim$family
#> [1] "lnorm"
#>
#>
#> $disease_threshold
#> [1] 20
#>
#> attr(,"class")
#> [1] "tsd_burden_levels"